In SQL, the SELECT and HAVING clauses serve different purposes in querying data from a database. Here's a brief overview of each:
SELECT Clause
Purpose: The SELECT clause is used to specify the columns that you want to retrieve from the database. It can also be used to perform calculations and apply functions to the data.
Usage:
Retrieve specific columns: SELECT column1, column2 FROM table;
Perform calculations: SELECT column1, SUM(column2) FROM table GROUP BY column1;
Use functions: SELECT column1, AVG(column2) FROM table GROUP BY column1;
HAVING Clause
Purpose: The HAVING clause is used to filter the results of a GROUP BY operation. It is similar to the WHERE clause, but WHERE cannot be used with aggregate functions. HAVING is applied after the aggregation is performed.
Usage:
Filter grouped results: SELECT column1, SUM(column2) FROM table GROUP BY column1 HAVING SUM(column2) > 100;
Apply conditions on aggregated data: SELECT column1, AVG(column2) FROM table GROUP BY column1 HAVING AVG(column2) < 50;
Key Differences
Timing of Execution:
SELECT is used to specify and manipulate the columns to be retrieved before grouping and aggregation.
HAVING is used to filter the results after the grouping and aggregation have taken place.
Use of Aggregate Functions:
SELECT can use aggregate functions to perform calculations on columns.
HAVING is specifically for filtering results based on conditions applied to aggregate functions.
Filtering:
WHERE (often used with SELECT) filters rows before any groupings are made.
HAVING filters groups after the GROUP BY clause has been applied.
Example
Here's an example to illustrate the use of both SELECT and HAVING:
SELECT ProductID, SUM(Quantity) AS TotalQuantity
FROM Sales
GROUP BY ProductID;
SELECT ProductID, SUM(Quantity) AS TotalQuantity
FROM Sales
GROUP BY ProductID
HAVING SUM(Quantity) > 100;
Select vs Having operation in SQL
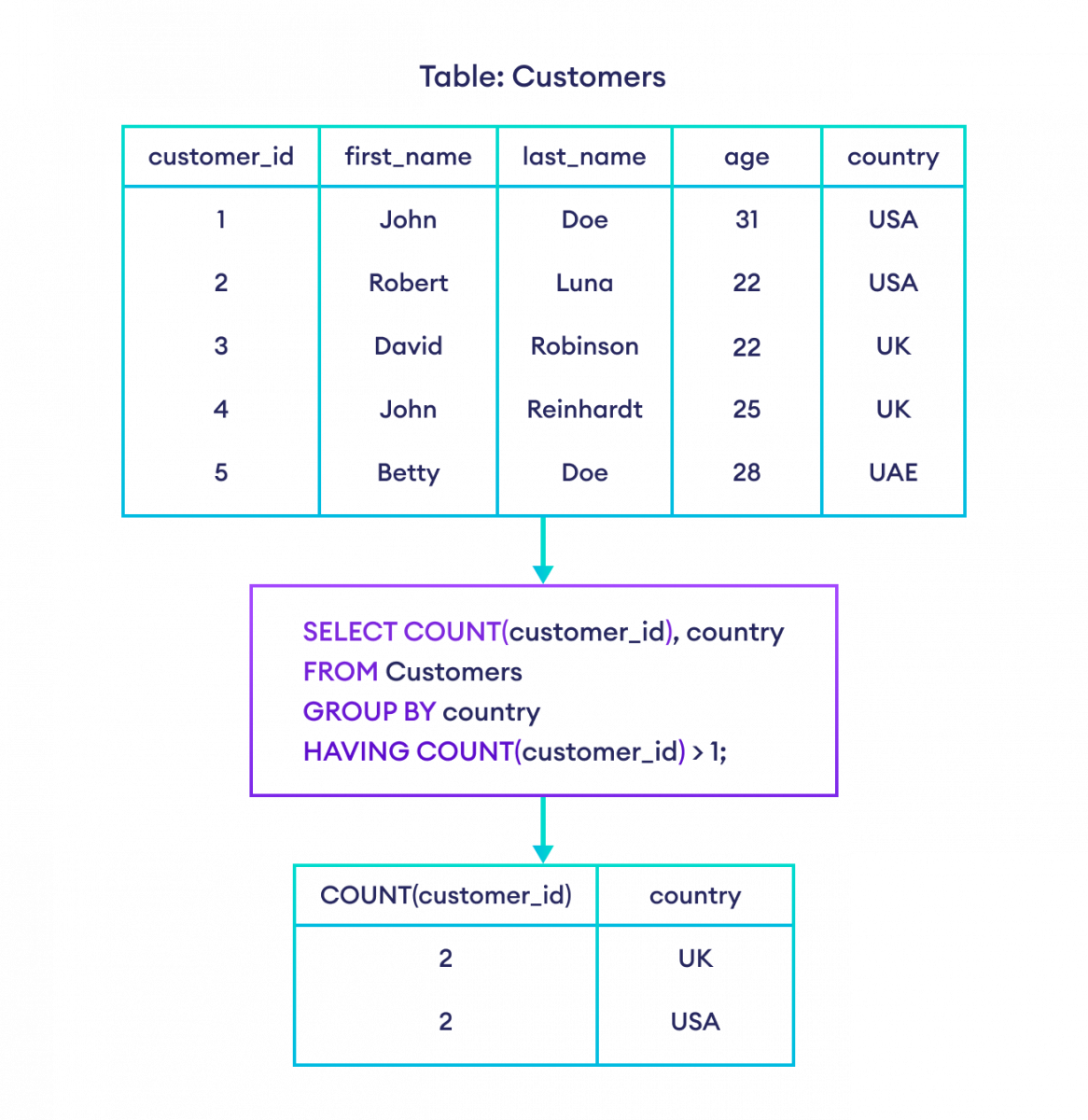