In SQL, the DELETE statement is used to remove one or more records from a table. The basic syntax for the DELETE statement is as follows:
DELETE FROM table_name
WHERE condition;
Here's what each part of the statement does:
DELETE FROM: This specifies that you want to delete records from a table.
table_name: This is the name of the table from which you want to delete records.
WHERE condition: This is an optional part of the statement that allows you to specify which records should be deleted based on certain conditions. If you omit the WHERE clause, all records from the table will be deleted.
Here's an example of using the DELETE statement to delete records from a hypothetical employees table where the department is 'HR':
DELETE FROM employees
WHERE department = 'HR';
This would delete all records from the employee's table where the department is 'HR'.
It's important to use the WHERE clause carefully with the DELETE statement to ensure that you only delete the records you intend to delete. If you omit the WHERE clause, you'll delete all records from the specified table, which can lead to data loss if done unintentionally.
More Example
1. Deleting a Single Record:
DELETE FROM employees
WHERE employee_id = 1001;
2. Deleting Multiple Records Based on a Condition:
DELETE FROM orders
WHERE order_date < '2023-01-01';
3. Deleting All Records from a Table:
DELETE FROM customers;
4. Deleting Records Using Subquery:
DELETE FROM products
WHERE category_id IN (SELECT category_id FROM categories WHERE category_name = 'Electronic');
Delete Operation In SQL
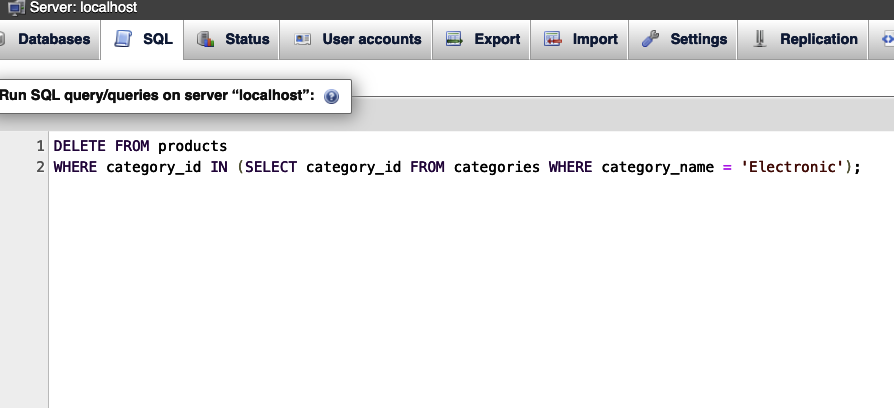